Hour of Code with EarSketch
Welcome to the EarSketch Hour of Code.
What is EarSketch?
EarSketch is a platform for making music with code. In this Hour of Code tutorial, you will learn the basics of coding in EarSketch and will be able to make music like this:
When you are done listening to the music, press the right arrow button at the bottom of the screen to continue.
EarSketch is a platform for making music with code. In this Hour of Code tutorial, you will learn the basics of coding in EarSketch and will be able to make music like this:
When you are done listening to the music, press the right arrow button at the bottom of the screen to continue.
Getting Started
Below is some code created in EarSketch. It is written in a programming language called Python. Click the blue icon in the upper right corner to copy the code into the code editor. Don’t worry about understanding the code yet.
from earsketch import *
setTempo(120)
# Add Sounds
fitMedia(RD_UK_HOUSE_MAINBEAT_8, 1, 1, 5)
fitMedia(RD_POP_PADCHORD_2, 2, 1, 9)
# Fills
fillA = "0---0-0-00--0000"
fillB = "0--0--0--0--0-0-"
fillC = "--000-00-00-0-00"
makeBeat(OS_SNARE03, 3, 4, fillA)
# More Sounds
# fitMedia(YG_RNB_TAMBOURINE_1,4,1,9)
# fitMedia(YG_FUNK_HIHAT_2,5,1,9)
# fitMedia(Y01_CRASH_1,6,1,9)
# fitMedia(YG_FUNK_CONGAS_3,7,1,9)
# fitMedia(RD_POP_ARPBASS_4,8,1,9)
# fitMedia(RD_POP_KEYPLUCK_4,9,1,9)
# fitMedia(RD_POP_SYNTHLEAD_1,10,1,9)
# fitMedia(RD_POP_TB303LEAD_3,11,1,9)
# fitMedia(RD_POP_SYNTHBASS_6,12,1,9)
# fitMedia(DUBSTEP_PERCDRUM_006,13,1,9)
Once the code has been copied to the code editor, click the blue "Import To Edit" button. This will allow you to make changes to the code.
Below is some code created in EarSketch. It is written in a programming language called JavaScript. Click the blue icon in the upper right corner to copy the code into the code editor. Don’t worry about understanding the code yet.
setTempo(120);
// Add Sounds
fitMedia(RD_UK_HOUSE_MAINBEAT_8, 1, 1, 5);
fitMedia(RD_POP_PADCHORD_2, 2, 1, 9);
// Fills
var fillA = "0---0-0-00--0000";
var fillB = "0--0--0--0--0-0-";
var fillC = "--000-00-00-0-00";
makeBeat(OS_SNARE03, 3, 4, fillA);
// More Sounds
// fitMedia(YG_RNB_TAMBOURINE_1,4,1,9);
// fitMedia(YG_FUNK_HIHAT_2,5,1,9);
// fitMedia(Y01_CRASH_1,6,1,9);
// fitMedia(YG_FUNK_CONGAS_3,7,1,9);
// fitMedia(RD_POP_ARPBASS_4,8,1,9);
// fitMedia(RD_POP_KEYPLUCK_4,9,1,9);
// fitMedia(RD_POP_SYNTHLEAD_1,10,1,9);
// fitMedia(RD_POP_TB303LEAD_3,11,1,9);
// fitMedia(RD_POP_SYNTHBASS_6,12,1,9);
// fitMedia(DUBSTEP_PERCDRUM_006,13,1,9);
Once the code has been copied to the code editor, click the blue "Import To Edit" button. This will allow you to make changes to the code.
Run The Code
Run the example code by pressing the green "Run" button at the top of the code editor.
After you run the code, you will see that the top section of the screen will be populated by rectangles. These represent sound clips.
Play the music that the code generated by clicking the play button above the sound clips.
The video below shows you how to open, import, run, and listen to the example code.
Run the example code by pressing the green "Run" button at the top of the code editor.
After you run the code, you will see that the top section of the screen will be populated by rectangles. These represent sound clips.
Play the music that the code generated by clicking the play button above the sound clips.
The video below shows you how to open, import, run, and listen to the example code.
Adding Sound Clips
Above the clips is a timeline which displays time in seconds (top) and measures (bottom). Measure is a musical term for a length of time.
The clips are organized in rows, called tracks in EarSketch.
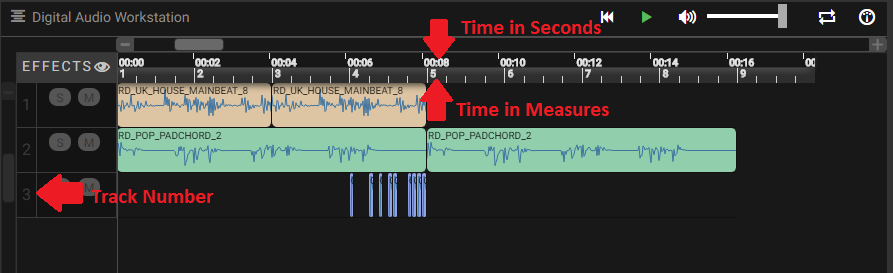
The code that adds the sound clips to the first track is on line 7 in the Code Editor.
Line 7 uses a function called fitMedia()
. A function is a piece of code that performs an operation. The fitMedia()
function adds a sound clip to the time line.
A function generally requires parameters in order to know precisely what you want to do. The fitMedia()
function takes in four input parameters:
-
sound clip
-
track number
-
start measure
-
end measure
Let’s look at line 7:
fitMedia(RD_UK_HOUSE_MAINBEAT_8,1,1,5)
This means the sound clip called "RD_UK_HOUSE_MAINBEAT_8" will be added to track 1, starting at measure 1 and ending at measure 5.
Above the clips is a timeline which displays time in seconds (top) and measures (bottom). Measure is a musical term for a length of time.
The clips are organized in rows, called tracks in EarSketch.
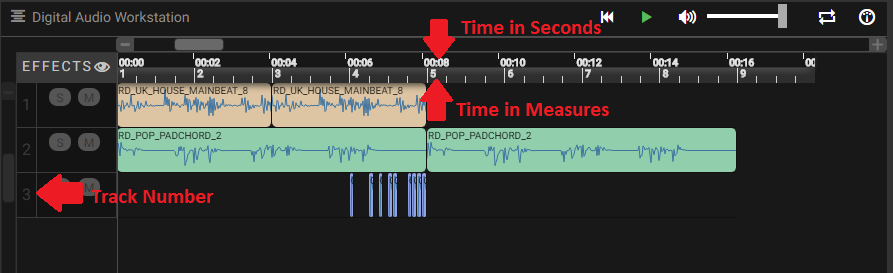
The code that adds the sound clips to the first track is on line 7 in the Code Editor.
Line 7 uses a function called fitMedia()
. A function is a piece of code that performs an operation. The fitMedia()
function adds a sound clip to the time line.
A function generally requires parameters in order to know precisely what you want to do. The fitMedia()
function takes in four input parameters:
-
sound clip
-
track number
-
start measure
-
end measure
Let’s look at line 7:
fitMedia(RD_UK_HOUSE_MAINBEAT_8,1,1,5);
This means the sound clip called "RD_UK_HOUSE_MAINBEAT_8" will be added to track 1, starting at measure 1 and ending at measure 5.
Changing the End Time of a Clip
Let’s change line 7 so the sound clip ends at measure 9 instead of 5. The line should now look like this:
fitMedia(RD_UK_HOUSE_MAINBEAT_8,1,1,9)
Use the code editor to change line 7. Then run the code, play the music, and listen to how it has changed.
Let’s change line 7 so the sound clip ends at measure 9 instead of 5. The line should now look like this:
fitMedia(RD_UK_HOUSE_MAINBEAT_8,1,1,9);
Use the code editor to change line 7. Then run the code, play the music, and listen to how it has changed.
Changing the Start Time of a Clip
Now let’s look at line 8. The line reads:
fitMedia(RD_POP_PADCHORD_2,2,1,9)
This means the sound clip called "RD_POP_PADCHORD_2" will be added to track 2, starting at measure 1 and ending at measure 9.
Change the line so the sound clip starts at measure 5 instead of 1. The line should now look like this:
fitMedia(RD_POP_PADCHORD_2,2,5,9)
Use the code editor to change line 8. Then run the code, play the music, and listen to how it has changed.
Now let’s look at line 8. The line reads:
fitMedia(RD_POP_PADCHORD_2,2,1,9);
This means the sound clip called "RD_POP_PADCHORD_2" will be added to track 2, starting at measure 1 and ending at measure 9.
Change the line so the sound clip starts at measure 5 instead of 1. The line should now look like this:
fitMedia(RD_POP_PADCHORD_2,2,5,9);
Use the code editor to change line 8. Then run the code, play the music, and listen to how it has changed.
Experiment with Start and End Times
Now that you know how to change the start and end times of clips in EarSketch, you can customize the music.
On lines 7 and 8, try some different values for the start and end parameters. Then, run the code and listen to how the music changes.
You can use whole numbers (like 1, 5, or 27) or decimals (like 1.25, 5.5, or 27.75) for start and end times. Remember that the smallest number you can use for a measure or track is 1.
Now that you know how to change the start and end times of clips in EarSketch, you can customize the music.
On lines 7 and 8, try some different values for the start and end parameters. Then, run the code and listen to how the music changes.
You can use whole numbers (like 1, 5, or 27) or decimals (like 1.25, 5.5, or 27.75) for start and end times. Remember that the smallest number you can use for a measure or track is 1.
Drum Fills
Line 14 of the code uses the makeBeat()
function. This function allows you to make custom rhythms.
The makeBeat()
function takes in four input parameters:
-
sound clip
-
track number
-
start measure
-
beat string
Line 14 contains the code:
makeBeat(OS_SNARE03, 3, 4, fillA)
This means the beat will use the sound clip "OS_SNARE03", will be added to track 3 at measure 4, and use the beat string fillA
.
Line 14 of the code uses the makeBeat()
function. This function allows you to make custom rhythms.
The makeBeat()
function takes in four input parameters:
-
sound clip
-
track number
-
start measure
-
beat string
Line 14 contains the code:
makeBeat(OS_SNARE03, 3, 4, fillA);
This means the beat will use the sound clip "OS_SNARE03", will be added to track 3 at measure 4, and use the beat string fillA
.
Variables
In this example, fillA
is a variable. Variables hold data such as numbers or words to be used later in the code.
The fillA
variable holds a string of characters, "0---0-0-00—0000"
, which describes a rhythm for makeBeat()
. Strings always begin and end with quotation marks. The code also defines the variables fillB
and fillC
as strings.
Let’s edit line 14 to use one of the other variables. Choose either fillB
or fillC
to replace fillA
in the code. Here is what line 14 will look like if you choose fillC
:
makeBeat(OS_SNARE03, 3, 4, fillC)
Once you have chosen a new drum fill, run the code and listen to the result.
In this example, fillA
is a variable. Variables hold data such as numbers or words to be used later in the code.
The fillA
variable holds a string of characters, "0---0-0-00—0000"
, which describes a rhythm for makeBeat()
. Strings always begin and end with quotation marks. The code also defines the variables fillB
and fillC
as strings.
Let’s edit line 14 to use one of the other variables. Choose either fillB
or fillC
to replace fillA
in the code. Here is what line 14 will look like if you choose fillC
:
makeBeat(OS_SNARE03, 3, 4, fillC);
Once you have chosen a new drum fill, run the code and listen to the result.
Editing Beat Strings
EarSketch has a function called reverseString()
which can reverse the order of the characters in a string.
On line 16 in the code, type the following:
fillRev = reverseString(fillA)
This creates a new variable called fillRev
that contains a reversed version of fillA
. Since fillA
is "0---0-0-00—0000"
, fillRev
will be "0000—00-0-0---0"
.
EarSketch has a function called reverseString()
which can reverse the order of the characters in a string.
On line 16 in the code, type the following:
fillRev = reverseString(fillA);
This creates a new variable called fillRev
that contains a reversed version of fillA
. Since fillA
is "0---0-0-00—0000"
, fillRev
will be "0000—00-0-0---0"
.
Making a New Fill
We can use fillRev
in a makeBeat()
function. Instead of editing the existing makeBeat()
function, we will make a new one to add a second drum fill to the music.
On line 17, type the following code to put the reversed fill into your song:
makeBeat(OS_SNARE03, 3, 8, fillRev)
This means the beat will use the sound clip "OS_SNARE03", will be added to track 3 at measure 8, and will use the beat string stored in fillRev
.
Run the code and listen to the result.
We can use fillRev
in a makeBeat()
function. Instead of editing the existing makeBeat()
function, we will make a new one to add a second drum fill to the music.
On line 17, type the following code to put the reversed fill into your song:
makeBeat(OS_SNARE03, 3, 8, fillRev);
This means the beat will use the sound clip "OS_SNARE03", will be added to track 3 at measure 8, and will use the beat string stored in fillRev
.
Run the code and listen to the result.
Add Your Own Fill
Use what you’ve learned to write another makeBeat()
call at a different measure with a different beat string.
You can use or modify one of the provided strings or try to write one from scratch using "0" and "-" characters. Beat strings are usually 16 characters long, which represents one measure of music.
Use what you’ve learned to write another makeBeat()
call at a different measure with a different beat string.
You can use or modify one of the provided strings or try to write one from scratch using "0" and "-" characters. Beat strings are usually 16 characters long, which represents one measure of music.
Using Comments
Throughout the example code, you’ll see section labels such as # Add Sounds
, # Fills
, and # More Sounds
. The "#" symbol at the start of the line means that line is a comment. Comments provide information about the code but are ignored by the computer.
Comments can be used as labels and explanations to help make code easier to read. They can also be used to disable lines of code so they are ignored by the computer when the code is run.
Throughout the example code, you’ll see section labels such as // Add Sounds
, // Fills
, and // More Sounds
. The "//" symbol at the start of the line means that line is a comment. Comments provide information about the code but are ignored by the computer.
Comments can be used as labels and explanations to help make code easier to read. They can also be used to disable lines of code so they are ignored by the computer when the code is run.
Adding More Sounds
Under the # More Sounds
comment, there is a series of fitMedia()
functions that are commented out. Each contains a different sound.
You can enable them by deleting the "#" character at the beginning of the line.
Try uncommenting different combinations of lines. Then, run the code and listen to the result. See if you can guess what each line of code will add to the music before you press run.
Under the // More Sounds
comment, there is a series of fitMedia()
functions that are commented out. Each contains a different sound.
You can enable them by deleting the "//" character at the beginning of the line.
Try uncommenting different combinations of lines. Then, run the code and listen to the result. See if you can guess what each line of code will add to the music before you press run.
Make Your Own Song
It is time to make your own music using EarSketch.
Comment and uncomment lines of code, change start and end times in fitMedia()
, and add fills with makeBeat()
.
You can edit or comment out functions that were included in the example code or try to write your own new lines of code. If you want to start a new song from scratch, press the +
icon to create a new Python script.
If you want to explore more sound clips, you can use the Sound Browser. Access the browser by clicking the icon on the left side of the screen.
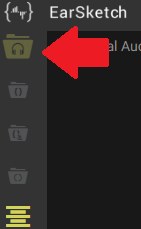
In the browser, you can search for and filter through all of the sounds available in EarSketch.
It is time to make your own music using EarSketch.
Comment and uncomment lines of code, change start and end times in fitMedia()
, and add fills with makeBeat()
.
You can edit or comment out functions that were included in the example code or try to write your own new lines of code. If you want to start a new song from scratch, press the +
icon to create a new JavaScript script.
If you want to explore more sound clips, you can use the Sound Browser. Access the browser by clicking the icon on the left side of the screen.
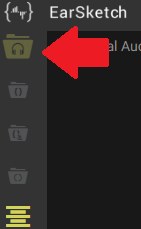
In the browser, you can search for and filter through all of the sounds available in EarSketch.
Going Further With EarSketch
EarSketch offers much more than we had time to cover in the last hour. There is a full length curriculum included in this panel. It can be accessed by clicking the Table of Contents icon at the top right of the browser window.
You can use the full curriculum to learn more about programming and create more complex music. The example below represents just one of the possibilities.
from earsketch import *
from random import randint
setTempo(120)
def addSection(clips, drums, beat, lead, segLen, start, length):
track = 3
for n in range(length):
makeBeat(drums, 1, start + n, beat)
for c in clips:
fitMedia(c, track, start, start + length)
track += 1
if lead:
generateLead(segLen, start, length)
def generateLead(segLen, start, L):
leadClips = [RD_EDM_RAVELEAD_1, RD_EDM_RAVELEAD_2, RD_EDM_RAVELEAD_3, RD_EDM_RAVELEAD_4, RD_EDM_RAVELEAD_5, RD_EDM_RAVELEAD_6]
numSegs = int(L / segLen)
for n in range(numSegs):
r = randint(0, 5)
fitMedia(leadClips[r], 2, start, start + segLen)
start += segLen
clips1 = [RD_EDM_RAZORBASS_2, RD_EDM_ANALOGLEAD_4, RD_EDM_ANALOGPLUCK_2]
clips2 = [RD_EDM_CHORDPART_5, RD_EDM_PERCSYNTHLEAD_1, YG_EDM_FX_12]
clips3 = [RD_EDM_CHORDPART_5, RD_EDM_PERCSYNTHLEAD_1, YG_EDM_FX_12, RD_EDM_SFX_RISER_1]
drums = [OS_KICK02, OS_SNARE04, Y24_FX_2, Y24_ELECTRO_2, OS_OPENHAT05]
beat1 = "0430322-12-13223"
beat2 = "0+1+0+110+1+0111"
beat3 = "0+++0+++0+++0+++"
addSection(clips2, drums, beat3, False, 1, 1, 4)
addSection(clips3, drums, beat2, True, 2, 5, 4)
addSection(clips1, drums, beat1, True, 0.5, 9, 4)
addSection(clips1, drums, beat1, True, 0.25, 13, 4)
addSection(clips2, drums, beat3, True, 1, 17, 4)
addSection(clips2, drums, "", False, 1, 21, 4)
# Effetcs
pan = [0, 0, 50, -50, -35, 35]
vol = [0, -1, 0, 0, 0, 0]
for i in range(6):
setEffect(i + 1, PAN, LEFT_RIGHT, pan[i])
setEffect(i + 1, VOLUME, GAIN, vol[i])
EarSketch offers much more than we had time to cover in the last hour. There is a full length curriculum included in this panel. It can be accessed by clicking the Table of Contents icon at the top right of the browser window.
You can use the full curriculum to learn more about programming and create more complex music. The example below represents just one of the possibilities.
setTempo(120);
function addSection(clips, drums, beat, lead, segLen, start, length) {
var track = 3;
for (var n = 0; n < length; n++) {
makeBeat(drums, 1, start + n, beat);
}
for (var c = 0; c < clips.length; c++) {
fitMedia(clips[c], track, start, start + length);
track += 1;
}
if (lead) {
generateLead(segLen, start, length);
}
}
function generateLead(segLen, start, L) {
var leadClips = [RD_EDM_RAVELEAD_1, RD_EDM_RAVELEAD_2, RD_EDM_RAVELEAD_3, RD_EDM_RAVELEAD_4, RD_EDM_RAVELEAD_5, RD_EDM_RAVELEAD_6];
var numSegs = Math.floor(L / segLen);
for (var n = 0; n < numSegs; n++) {
var r = Math.floor(Math.random() * 6);
fitMedia(leadClips[r], 2, start, start + segLen);
start += segLen;
}
}
var clips1 = [RD_EDM_RAZORBASS_2, RD_EDM_ANALOGLEAD_4, RD_EDM_ANALOGPLUCK_2];
var clips2 = [RD_EDM_CHORDPART_5, RD_EDM_PERCSYNTHLEAD_1, YG_EDM_FX_12];
var clips3 = [RD_EDM_CHORDPART_5, RD_EDM_PERCSYNTHLEAD_1, YG_EDM_FX_12, RD_EDM_SFX_RISER_1];
var drums = [OS_KICK02, OS_SNARE04, Y24_FX_2, Y24_ELECTRO_2, OS_OPENHAT05];
var beat1 = "0430322-12-13223";
var beat2 = "0+1+0+110+1+0111";
var beat3 = "0+++0+++0+++0+++";
addSection(clips2, drums, beat3, false, 1, 1, 4);
addSection(clips3, drums, beat2, true, 2, 5, 4);
addSection(clips1, drums, beat1, true, 0.5, 9, 4);
addSection(clips1, drums, beat1, true, 0.25, 13, 4);
addSection(clips2, drums, beat3, true, 1, 17, 4);
addSection(clips2, drums, "", false, 1, 21, 4);
// Effects
var pan = [0, 0, 50, -50, -35, 35];
var vol = [0, -1, 0, 0, 0, 0];
for (var i = 0; i < 6; i++) {
setEffect(i + 1, PAN, LEFT_RIGHT, pan[i]);
setEffect(i + 1, VOLUME, GAIN, vol[i]);
}: