Getting Started with EarSketch
This chapter covers the basic functionality of EarSketch. It explains the layout of the site and how to navigate through the various workspace panes. Most importantly, it provides tools for making a piece of music!
Why Learn Programming for Music?
Computers have greatly expanded the possibilities for getting involved in music. The musician’s toolbox has grown, and new skills are needed to use these tools. Programming involves creativity, so it fits well with making music. You’ll learn to think in both a structured and creative way, which is a valuable combination.
Programming experience also opens the door to many great and lucrative careers. Whether you’re interested in biology, physics, finance, math, robotics, education, making games, graphic design, music, literature, chemistry, or any other field, knowing how to program will help you become more well-rounded. Most importantly, anyone (including you) can learn to program. Like learning a musical instrument, it takes consistent practice to make progress. Don’t get discouraged if you get stuck, this is part of the process; ask for help in your class or look online.
In EarSketch, you will make music by writing algorithms, or code that the computer understands as a set of instructions. Here are some reasons you might want to program to create music:
-
You can automate tedious tasks. Imagine that you want to combine hundreds or even thousands of snippets of sound taken from dozens of audio files. You can do this through a graphical user interface (GUI) by manually clicking and dragging audio files, but it could take many hours. You can create the same music in much less time if you can efficiently describe what you want to do through a computer program.
-
You can experiment easily. What if the song you created out of thousands of audio segments isn’t perfect? You may want to tweak it to make it better. Some changes, like adjusting volume, are easy to make with a GUI. Other changes, like swapping out every occurrence of an audio file for a new one, are tedious to implement with a graphical interface. Through programming, you can quickly explore these "what if" questions by changing just one or two lines of code.
-
You can roll the dice. Algorithmic composition has a long history of using the computer to make random decisions, like flipping a coin or rolling dice. One reason for doing this is to make the music sound more human. For example, you might randomly change the starting time of an audio clip by a tiny amount each time it is repeated, in order to simulate the imperfect timing of humans. Likewise, you might randomly choose among 5 different variations of a drum beat in each measure of music, so that the drum track always sounds just a little bit different.
Here’s an example of some music made in EarSketch. This example is in an electronic dance music style, but you can make any kind of music you like.
Tools of the Trade: DAWs and APIs
The Digital Audio Workstation, or DAW, is the main tool for producing music on a computer. A DAW is specialized computer software for recording, editing, and playing digital audio files. In the context of a DAW, these audio files are called clips. The DAW allows you to edit and combine multiple clips simultaneously on a musical timeline, making it easy to synchronize and visualize different parts. DAWs are used in both professional recording studios and in home laptop-based studios. Some popular DAWs include Pro Tools and Logic Pro, GarageBand, and Reaper.
So what exactly is EarSketch? EarSketch is a DAW with extra features: the ability to place audio clips into a DAW timeline using computer code. This opens up musical possibilities that are difficult or impossible to create with a regular DAW, and makes many tasks much faster. In addition to adding extra features to a DAW, EarSketch adds features to a programming language. Programming languages come with a set of built-in tools, most of which are general-purpose. EarSketch adds extra tools to this set to help us accomplish our specific goal of making music. This collection of tools is called an Application Programming Interface, or API. Other examples of APIs include the Google Maps API (a set of tools for embedding maps into websites or apps) and the YouTube API (tools for embedding YouTube videos in websites).
The EarSketch Workspace
Here are the different sections of the EarSketch workspace:
-
Accounts (top right): Log in, create an account, or reset your password.
-
Sound Browser: Browse or search 4,000 audio clips to use in your music, made by musicians/producers Young Guru and Richard Devine. You can also record your own sounds or upload sound files you already have.
-
Scripts Browser: When you write code in EarSketch, your scripts are automatically saved to the EarSketch server. Go here to find your saved scripts, open them, export them as WAV or MP3 audio files, and share them with others.
-
Share Browser: When you open an EarSketch script shared with you by someone else, this will show you information about the script and additional options.
-
API Browser: Information on every EarSketch function.
-
Digital Audio Workstation (DAW): A timeline view of your current song, showing which audio clips you have added to the song and when they come in. It lets you hear your song and visualize its structure.
-
Code Editor: A text editor with numbered lines. Type your code here, press "Run", and it will turn into music in the DAW.
-
Console: The console displays important information about your code as it runs, including the location of errors in your code. It is a common and important feature in programming tools.
-
Curriculum: The current active panel. Here, you can learn how to make music with code.
Running a Script
The basic workflow for making a song in EarSketch follows: type your musical code into the code editor, press the run button to execute the code and add the music to the DAW, and press play in the DAW to hear it.
Take a look at the bar just above the Code Editor panel. There are some useful buttons and menus here: Run and Options (the gear icon).
When we write computer programs in EarSketch, we use the Python or JavaScript programming language. You are in Python mode. You can select the language you prefer each time you create a new script by clicking on "+" or "create a new script." Python is a general-purpose computer programming language that is used by many large companies, and is one of the ten most popular programming languages in the world. Python has been used for web development by companies like Yahoo and Google. In addition to web development, Python can be used for game development, science, education, and graphics. Games that have been created with Python include Civilization 4, Battlefield 2, and Crystal Space. Other well-known companies that have made extensive use of the Python programming language include The National Weather Service, NASA, IBM, Disney, and Nokia.
When we write computer programs in EarSketch, we use the Python or JavaScript programming language. You are in JavaScript mode. You can select the language you prefer each time you create a new script by clicking on "+" or "create a new script." JavaScript is one of the ten most popular programming languages in the world. It is primarily used in web development, but it is also widely used for many other purposes, such as animation and interface enhancements. Almost every website uses JavaScript.
Let’s try running a code example in EarSketch! Take a look at the box of text below; this is our code example. Press the blue clipboard icon in the top right to paste the example into the code editor. Don’t worry about understanding the code at this point, we will learn its meaning later.
# Intro Script: This code adds one audio clip to the DAW
# Setup Section
from earsketch import *
setTempo(120)
# Music Section
fitMedia(TECHNO_SYNTHPLUCK_001, 1, 1, 9)
// Intro Script: This code adds one audio clip to the DAW
// Setup Section
setTempo(120);
// Music Section
fitMedia(TECHNO_SYNTHPLUCK_001, 1, 1, 9);
Python and Javascript are both scripting languages, so your code is called a script. To turn your code into music, press the Run button (above the code editor). You should see some changes in the DAW and the Console. When you press run, all of the instructions in your code are carried out. In the DAW, press the play button to hear the music.
Adding Comments
Let’s make a small modification to the current project. We’ll add our name to the project. On lines 1-10, notice that each line starts with a pound sign: #. The computer does not execute a line of code that is preceded by #. This is called a comment. Comments are used by programmers to make notes on their code for them or other programmers to read later. On line 5 of the previous example, type your name to the right of "author:".
Let’s make a small modification to the current project. We’ll add our name to the project. On lines 1-10, notice that each line starts with two forward-slashes: //
. The computer does not execute a line of code that is preceded by //
. This is called a comment. Comments are used by programmers to make notes on their code, for them or other programmers to read later. On line 5 of the previous example, to the right of "author:" type your name.
The DAW in Detail
Take a look at the DAW. The DAW consists of several items:
-
Playhead: The red line, which represents your playback location in the timeline. The play button will start playback at the playhead’s location.
-
Transport Controls: The buttons at the top right of the DAW. You’ve probably seen most of these in a media player like iTunes. From left to right, the buttons are:
-
Reset: Press to jump the playhead back to the beginning.
-
Play/Pause: Press this to hear the music you’ve added. Playback begins at the playhead.
-
Mute: Toggles sound on and off.
-
Volume Slider: Adjust the volume of playback by moving the slider.
-
Loop: When the playhead reaches the end of the timeline, automatically start playing from the beginning again.
-
Toggle Metronome: Play a click track over your music.
-
-
Measure Numbers: At the top of the DAW timeline, there is a horizontal series of whole numbers. If this were a normal timeline, the numbers would represent minutes and seconds; however, here they represent measure numbers. A measure is a unit of musical time that depends on the speed (a.k.a. tempo) of a song. The tempo has to be specified in every script. For now, think of a measure as a block of time. This is how we tell EarSketch where to place our audio clips. Click on a measure number to move the playhead to it.
-
Audio Clips: If you have added music to the DAW, the DAW should display some boxes with squiggly lines inside. These are audio clips. They provide a visual representation of the sounds they contain.
-
Tracks: Every audio clip is placed on a specific track. Tracks are the rows that run across the DAW; they are numbered on the left. Tracks help you organize your sounds by instrument-type; for example, you would record each instrument (vocals, lead guitar, rhythm guitar, bass, drums, etc.) on a separate track in a recording studio. You can only have one audio clip at a given time on each track. So, having multiple tracks also means you can overlap them.
-
Effects Toggle: Show or hide the effects added on each track, if any. Note that the effects will still play back; the toggle is just for visuals.
-
Solo/Mute: Next to each track number, the "S" and "M" stand for solo and mute. Mute turns off playback for that track, and Solo turns off playback for all other tracks.
What is Programming?
A computer program is a sequence of instructions that the computer executes. It is used to accomplish a specific task or set of tasks. Programming is the process of designing, writing, testing, debugging, and maintaining the code of computer programs. This code can be written in a wide variety of computer programming languages. Some of these languages include Java, C, Python, and JavaScript.
Programming languages consist of a collection of words and symbols that the computer can understand, and a syntax for organizing them. You can think of this like the vocabulary and syntax of spoken language. At the deepest level, computers operate in combinations of 1s and 0s: binary numbers. Thankfully, we don’t have to write programs in binary, as it would be very hard for us to understand! Just as a human might translate from English to French, the computer can translate human-readable programming languages into binary code.
Computer programs implement algorithms; in other words, a computer program describes a set of instructions for a computer to follow. We can think of the different lines of our code as being individual instructions that we give to the computer. The computer follows these instructions explicitly to execute our written code.
Programs are developed for a wide variety of purposes. In EarSketch, our goal in developing programs is creative musical expression. Computer programs can be built to deal with many kinds of inputs and outputs, such as tactile or visual information. In EarSketch, we focus on creating output in the form of digital audio, which you can listen to in the browser or save to your computer.
Sections of an EarSketch Script
In EarSketch, we will structure all of our sample projects in roughly the same way: as 4 sections marked by comments, each with a different purpose:
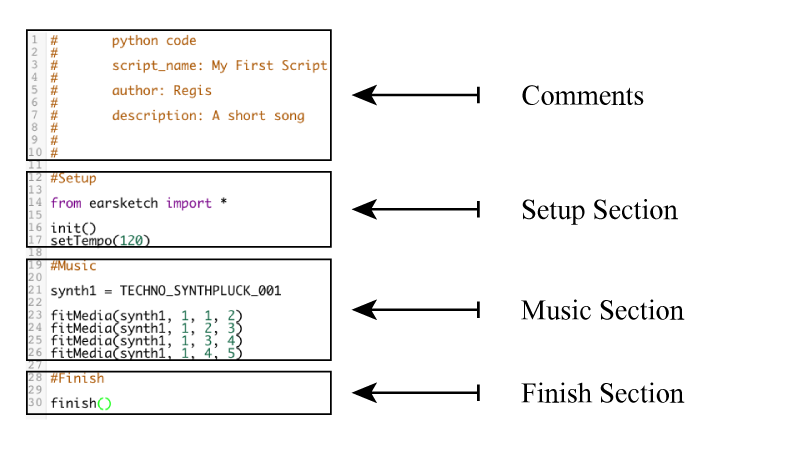
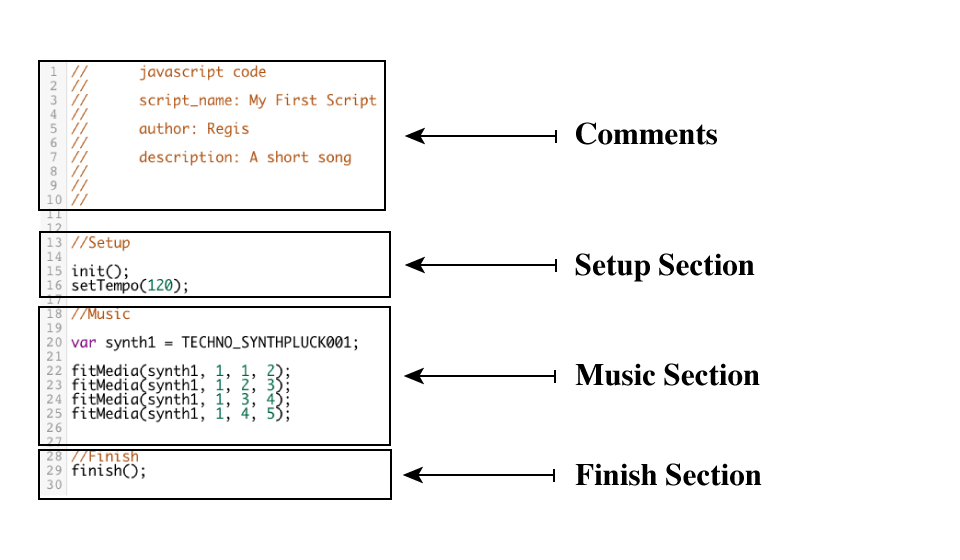
-
Comments Section
-
You can use comments anywhere in your code, but a block at the top is usually used to describe the whole project.
-
-
Setup Section
-
This code tells the DAW how to prepare to make music.
setTempo()
allows you to choose a tempo for the project, which can be anywhere from 45 to 220 beats per minute.from earsketch import *
adds the EarSketch API to the project. Every project with music in it must have these parts in the setup section.
-
-
Music Section
-
The most important section. All of the details of your composition go here.
-
-
Comments Section
-
You can use comments anywhere in your code, but a block at the top is usually used to describe the whole project.
-
-
Setup Section
-
This code tells the DAW how to prepare to make music.
setTempo()
allows you to choose a tempo for the project. Every project with music in it should have this in the setup section.
-
-
Music Section
-
The most important section. All of the details of your composition go here.
-
Creating a New Script
The following steps walk through how to create a new script in EarSketch.
-
Sign in or create a new account: We suggest you sign in to an EarSketch account before creating a new script. If you already have an EarSketch account, type your credentials into the "Username" and "Password" fields in the top right corner of your browser window. Then, click the arrow button to sign in. See the first figure at the end of this step for help.
If you don’t have an EarSketch account, it’s easy to create one! At the top right corner of your browser window, click the white button that reads "Create / Reset Account". See the first figure below. Next, select "Register a New Account" (you can also recover your password here if you forgot it). The "Create an account" dialogue box will open. This dialogue box is shown in the second figure below. Type your information into each field. You can choose your own username and password - just make sure you can remember them! Finally, click the "Create Account" button.
Figure 1.9.1: Login and create account buttonsFigure 1.9.2: Create an account dialogue box -
Click to create: If you don’t have any scripts open (i.e. the code editor is empty), you will see a large blue link in the middle of the code editor. This is shown in the figure below. Click that link to proceed.
Figure 1.9.3: Create a new script, empty script browserIf you have a script open already, then click on the "+" icon in the top right of the code editor. The icon’s location is shown in the figure below.
Figure 1.9.4: Create a new script, open scripts -
Choose a name and language: When the "Create a new script" dialog box opens (shown in the figure below), give your new script a descriptive name and select your preferred programming language (Python or Javascript). Finally, click the "Create" button.
Figure 1.9.5: The four sections of an EarSketch scriptFigure 1.9.5: The four sections of an EarSketch script -
Fill in the comments section: After your new script has been created, take a minute to fill in the comments section. Give your script an appropriate title, add your name, and write a short description of what it will do.
Composing In EarSketch
Let’s make some music with the script we just created. If you missed how to make a new script, jump back a section. Otherwise, follow these steps:
-
Add fitMedia(): To add a sound clip to the DAW, we start by typing
fitMedia()
.By itself,
fitMedia()
doesn’t do anything; we also need to specify the clip name to add to our DAW, the track number to place the clip on, and the starting and ending points of our clip. We will now specify these details between the parentheses offitMedia()
. -
Choose a clip and paste it into fitMedia(): Open the sound browser. Listen to a few clips using the play button and pick one you like. We want to paste this clip’s name into
fitMedia()
. Click so that your cursor is inside offitMedia()
’s parentheses. Back in the sound browser, click the paste button (clipboard) next to your chosen clip. You should now have something likefitMedia(Y18_DRUM_SAMPLES_2)
in your script. -
Choose a track and start/end points:
fitMedia()
still needs a bit more information to do anything:-
After the clip name in the parentheses, type a comma, then the track number:
1
. -
Type another comma, then a start measure:
1
. -
Type another comma, then the end measure:
5
.You should now have something like this:
fitMedia(Y18_DRUM_SAMPLES_1, 1, 1, 5)
. A more general way to think about this is asfitMedia(clipName, trackNumber, startMeasure, endMeasure)
. Note that every piece of information in the parentheses MUST be separated by commas.
-
-
Press Run and Play: Press Run to execute the script. Your chosen clip should be added to the DAW. Press play in the DAW and have a listen! If something isn’t working, take a look at our example below and compare with your code:
-
Add fitMedia(): To add a sound clip to the DAW, we start by typing
fitMedia()
.By itself,
fitMedia()
doesn’t do anything; we also need to specify the clip name to add to our DAW, the track number to place the clip on, and the starting and ending points of our clip. We will now specify these details between the parentheses offitMedia()
. -
Choose a clip and paste it into fitMedia(): Open the sound browser. Listen to a few clips using the play button and pick one you like. We want to paste this clip’s name into
fitMedia()
. Click so that your cursor is inside offitMedia()
’s parentheses. Back in the sound browser, click the paste button (clipboard) next to your chosen clip. You should now have something likefitMedia(Y18_DRUM_SAMPLES_2)
in your script. -
Choose a track and start/end points:
fitMedia()
still needs a bit more information to do anything:-
After the clip name in the parentheses, type a comma, then the track number:
1
. -
Type another comma, then a start measure:
1
. -
Type another comma, then the end measure:
5
.You should now have something like this:
fitMedia(Y18_DRUM_SAMPLES_1, 1, 1, 5)
. A more general way to think about this is asfitMedia(clipName, trackNumber, startMeasure, endMeasure)
. Note that every piece of information in the parentheses MUST be separated by commas.
-
-
Press Run and Play: Press Run to execute the script. Your chosen clip should be added to the DAW. Press play in the DAW and have a listen! If something isn’t working, take a look at our example below and compare with your code:
# Using fitMedia(): Adding a clip to the DAW
# Setup
from earsketch import *
setTempo(120)
# Music
fitMedia(Y18_DRUM_SAMPLES_2, 1, 1, 5)
// Using fitMedia(): Adding a clip to the DAW
// Setup
setTempo(120);
// Music
fitMedia(Y18_DRUM_SAMPLES_2, 1, 1, 5);
For an extra challenge, add more fitMedia()
calls to your script like we do below. Notice that we use a different track number for each fitMedia()
call:
# Using fitMedia() 2: Multiple fitMedia() calls on different tracks
# Setup Section
from earsketch import *
setTempo(100)
# Music Section
fitMedia(Y01_DRUMS_1, 1, 1, 9)
fitMedia(Y11_BASS_1, 2, 1, 9)
fitMedia(Y11_GUITAR_1, 3, 1, 9)
// Using fitMedia() 2: Multiple fitMedia() calls on different tracks
// Setup Section
setTempo(100);
// Music Section
fitMedia(Y01_DRUMS_1, 1, 1, 9);
fitMedia(Y11_BASS_1, 2, 1, 9);
fitMedia(Y11_GUITAR_1, 3, 1, 9);
Chapter 1 Summary
-
An algorithm is a set of instructions to be understood and carried out by the computer. Algorithms are written with computer code.
-
DAW’s are specialized computer software for recording, editing, and playing digital audio files, or clips. EarSketch is a DAW that allows audio clips to be placed on a timeline with code. EarSketch’s extra functionality is implemented using an API, a set of tools to accomplish special programming tasks.
-
EarSketch is a scripting language, so the code written within it is called a script.
-
To make music in EarSketch, code is first typed into the code editor panel. After pressing run, music is played in the DAW panel.
-
Sound clips can be found in the Sound Browser. They are referred to be typing or pasting their name in all caps.
-
Comments are lines of code that are not executed by the computer. However, they are useful for making notes within a script.
-
A computer program is a sequence of instructions that the computer executes to accomplish a specific task. Furthermore, programming is the process of designing, writing, testing, debugging, and maintaining code within a program.
-
Programming languages are a collection of words and symbols that are understood by the computer. A programming language follows a syntax in order to organize code.
-
An EarSketch script consists of a comments section, setup section, and music section.
-
from earsketch import *
adds the EarSketch API to your project. It must be included at the top of every script. -
setTempo()
lets you specify the tempo of your song. It must be included in every EarSketch script. -
Create a new script by clicking the large blue link or the "+" icon if another script is already open.
-
fitMedia()
is the primary way of adding sound to the DAW. It has four arguments, the information it needs to make music:-
fileName: The sound clip that is placed in the DAW.
-
trackNumber: The track on which music is placed.
-
startLocation: The measure at which the sound clip will start.
-
endLocation: The measure at which the sound clip will end.
-
-
An algorithm is a set of instructions to be understood and carried out by the computer. Algorithms are written with computer code.
-
DAW’s are specialized computer software for recording, editing, and playing digital audio files, or clips. EarSketch is a DAW that allows audio clips to be placed on a timeline with code. EarSketch’s extra functionality is implemented using an API, a set of tools to accomplish special programming tasks.
-
EarSketch is a scripting language, so the code written within it is called a script.
-
To make music in EarSketch, code is first typed into the code editor panel. After pressing run, music is played in the DAW panel.
-
Sound clips can be found in the Sound Browser. They are referred to be typing or pasting their name in all caps.
-
Comments are lines of code that are not executed by the computer. However, they are useful for making notes within a script.
-
A computer program is a sequence of instructions that the computer executes to accomplish a specific task. Furthermore, programming is the process of designing, writing, testing, debugging, and maintaining code within a program.
-
Programming languages are a collection of words and symbols that are understood by the computer. A programming language follows a syntax in order to organize code.
-
An EarSketch script consists of a comments section, setup section, and music section.
-
setTempo()
lets you specify the tempo of your song. It must be included in every EarSketch script. -
Create a new script by clicking the large blue link or the "+" icon if another script is already open.
-
fitMedia()
is the primary way of adding sound to the DAW. It has four arguments, the information it needs to make music:-
fileName: The sound clip that is placed in the DAW.
-
trackNumber: The track on which music is placed.
-
startLocation: The measure at which the sound clip will start.
-
endLocation: The measure at which the sound clip will end.
-
Questions
Which of the following is not true regarding APIs?"
-
They allow you to use Ableton Live within EarSketch.
-
They let different software components talk to one another.
-
They allow you to simplify complex coding tasks.
-
They often come as a collection, or library.
fitMedia()
is an example of what?
-
A Function
-
A Comment
-
A DAW
-
A Library